
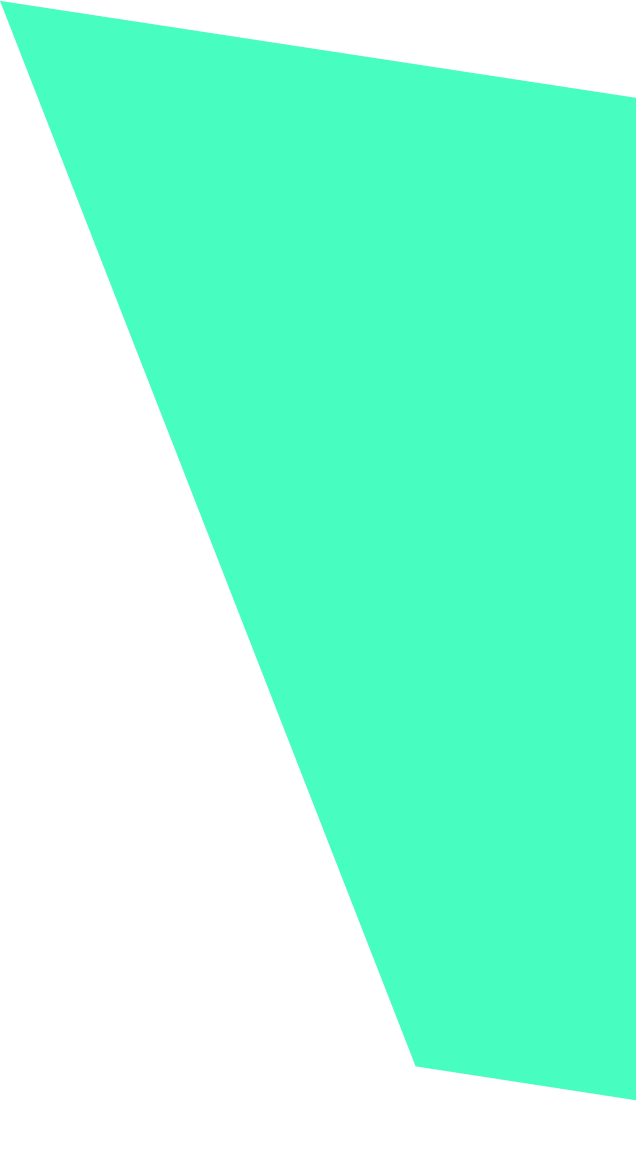
Tessell for Milvus
Create LLM apps, like TessellGPT with a low-code platform built on top of a fully managed service for Milvus, the world’s most powerful vector database purpose-built for AI workloads.
How Tessell for Milvus works?
Fully managed Milvus vector database service with enterprise-grade data management, performance optimized for production, cost-optimized for development, and all with your own security and compliance posture.
Store, index, and manage massive embedding vectors generated by deep neural networks and other machine learning (ML) models. Tessell for Milvus is highly resilient and reliable.
Where can vector databases help?
Retrieval Augmented Generation (RAG)
Build LLM apps with foundational LLM model using RAG.
Recommender System
Get personalized recommendations based on user profiles, behaviors, and queries, identifying vectors aligned with their interests.
Text/Semantic Search
Allow search engines to return results semantically similar to the query, even if they don't contain the exact keywords.
Image Similarity Search
Explore visually akin images within an extensive repository of image libraries.
Question Answering System
Build a chatbot for interactive question-answering that automatically responds to user queries.
Build LLM apps with Tessell for Milvus
Build your LLM apps in minutes with Tessell for Milvus. Tessell manages and provisions your vector databases, seamlessly integrates with popular LLM frameworks, ensuring smooth data flow and efficient model training, and powers powerful vector search capabilities for lightning-fast retrieval of similar text, images, or code within your LLM app.
1
2from pymilvus import connections, db
3from pymilvus import CollectionSchema, FieldSchema, DataType
4
5#Create client connection to Tessell for Milvus
6
7TESSELL_MILVUS_URI = "xxxxxxx.tessell.com:19530"
8
9conn = connections.connect(
10 alias="default",
11 uri=TESSELL_MILVUS_URI,
12 token="root:Milvus",
13 )
14
15db = conn.create_database('tessell_articles')
16
17article_id = FieldSchema(
18 name="article_id",
19 dtype=DataType.INT64,
20 is_primary=True,
21)
22article_title = FieldSchema(
23 name="article_title",
24 dtype=DataType.VARCHAR,
25 max_length=500,
26 # The default value will be used if this field is left empty during data inserts or upserts.
27 # The data type of `default_value` must be the same as that specified in `dtype`.
28 default_value="Unknown"
29)
30
31article_content= FieldSchema(
32 name="article_title",
33 dtype=DataType.VARCHAR,
34 max_length=10000,
35 # The default value will be used if this field is left empty during data inserts or upserts.
36 # The data type of `default_value` must be the same as that specified in `dtype`.
37 default_value="Unknown"
38)
39embeddings = FieldSchema(
40 name="book_intro",
41 dtype=DataType.FLOAT_VECTOR,
42 dim=786
43)
44schema = CollectionSchema(
45 fields=[article_id, article_title, article_content, embeddings],
46 description="Tessell articles knowledge basae",
47 enable_dynamic_field=True
48)
49collection_name = "tessell_articles"
1package main
2
3import (
4 "context"
5 "fmt"
6 "log"
7 "math/rand"
8 "time"
9
10 "github.com/milvus-io/milvus-sdk-go/v2/client"
11 "github.com/milvus-io/milvus-sdk-go/v2/entity"
12)
13
14func main() {
15 collectionName := "tessell_articles"
16 uri := "xxxxxxx.tessell.com:19530"
17 user := "username"
18 password := "password"
19
20 // connect to milvus
21 fmt.Println("Connecting to DB: ", uri)
22 ctx, cancel := context.WithCancel(context.Background())
23 defer cancel()
24
25 client, err := client.NewClient(ctx, client.Config{
26 Address: uri,
27 Username: user,
28 Password: password,
29 })
30
31 if err != nil {
32 log.Fatal("fail to connect to milvus", err.Error())
33 }
34 fmt.Println("Success!")
35
36 // delete collection if exists
37 has, err := client.HasCollection(ctx, collectionName)
38 if err != nil {
39 log.Fatal("fail to check whether collection exists", err.Error())
40 }
41 if has {
42 client.DropCollection(ctx, collectionName)
43 }
44
45 // create a collection
46 fmt.Println("Creating example collection: Tessell Articles")
47 schema := entity.NewSchema().WithName(collectionName).WithDescription("All articles published on tessell website.").
48 WithField(entity.NewField().WithName("article_id").WithDataType(entity.FieldTypeInt64).WithIsPrimaryKey(true).WithDescription("customized primary id")).
49 WithField(entity.NewField().WithName("article_title").WithDataType(entity.FieldTypeInt64).WithDescription("Article Title")).
50 WithField(entity.NewField().WithName("embeddings").WithDataType(entity.FieldTypeFloatVector).WithDim(128))
51
52 err = client.CreateCollection(ctx, schema, entity.DefaultShardNumber)
53 if err != nil {
54 log.Fatal("failed to create collection", err.Error())
55 }
56 fmt.Println("Success!")
57
58 // insert data
59 fmt.Println("Inserting 100000 entities... ")
60 dim := 128
61 num_entities := 100000
62 idList, countList := make([]int64, 0, num_entities), make([]int64, 0, num_entities)
63 vectorList := make([][]float32, 0, num_entities)
64 for i := 0; i < num_entities; i++ {
65 idList = append(idList, int64(i))
66 countList = append(countList, int64(i))
67 vec := make([]float32, 0, dim)
68 for j := 0; j < dim; j++ {
69 vec = append(vec, rand.Float32())
70 }
71 vectorList = append(vectorList, vec)
72 }
73
74 idData := entity.NewColumnInt64("article_id", idList)
75 countData := entity.NewColumnInt64("article_title", countList)
76 vectorData := entity.NewColumnFloatVector("embeddings", dim, vectorList)
77
78 begin := time.Now()
79 _, err = client.Insert(ctx, collectionName, "", idData, countData, vectorData)
80 if err != nil {
81 log.Fatal("fail to insert data", err.Error())
82 }
83 fmt.Println("Succeed in", time.Since(begin))
84
85 // create index
86 fmt.Println("Building AutoIndex...")
87 index, err := entity.NewIndexAUTOINDEX(entity.L2)
88 if err != nil {
89 log.Fatal("fail to get auto index", err.Error())
90 }
91 begin = time.Now()
92 err = client.CreateIndex(ctx, collectionName, "article_id", index, false)
93 if err != nil {
94 log.Fatal("fail to create index", err.Error())
95 }
96 fmt.Println("Succeed in", time.Since(begin))
97
98 // load collection
99 fmt.Println("Loading collection...")
100 begin = time.Now()
101 err = client.LoadCollection(ctx, collectionName, false)
102 if err != nil {
103 log.Fatal("fail to load collection", err.Error())
104 }
105 fmt.Println("Succeed in", time.Since(begin))
106
107 // search
108 sp, _ := entity.NewIndexAUTOINDEXSearchParam(1)
109 vectors := []entity.Vector{entity.FloatVector(vectorList[1])}
110 fmt.Println("Search...")
111 begin = time.Now()
112 searchResult, err := client.Search(
113 ctx,
114 collectionName, // collectionName
115 nil, // partitionNames
116 "", // expression
117 []string{"article_id"}, // outputFields
118 vectors, // vectors
119 "embeedings", // vectorField
120 entity.L2, // metricType
121 10, // topK
122 sp, // search params
123 )
124 if err != nil {
125 log.Fatal("search failed", err.Error())
126 }
127 fmt.Println("Succeed in", time.Since(begin))
128
129 for _, sr := range searchResult {
130 fmt.Println("ids: ", sr.IDs)
131 fmt.Println("Scores: ", sr.Scores)
132 }
133}
Integrates seamlessly with your tech stack
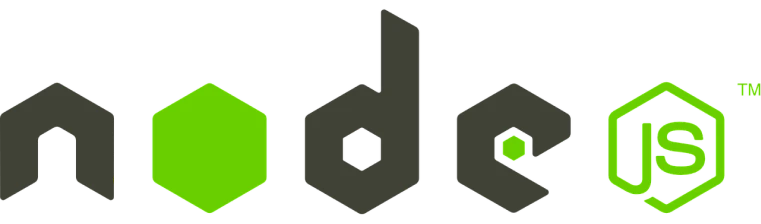

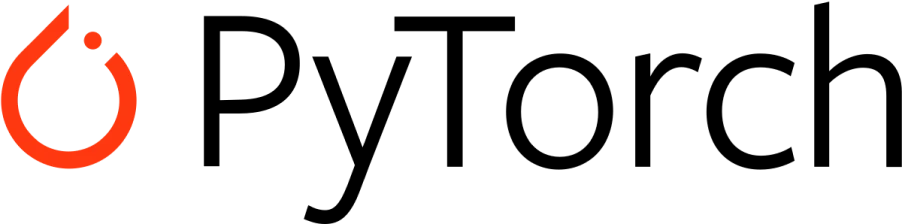


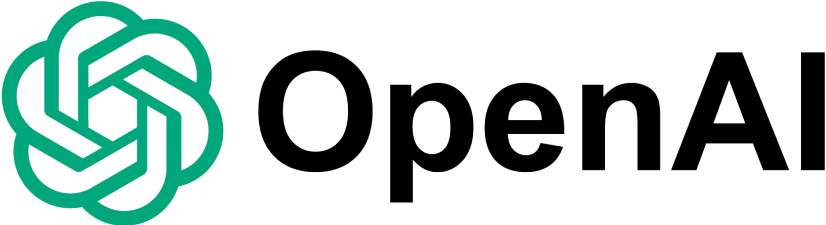
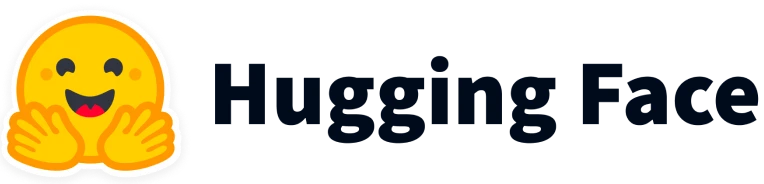
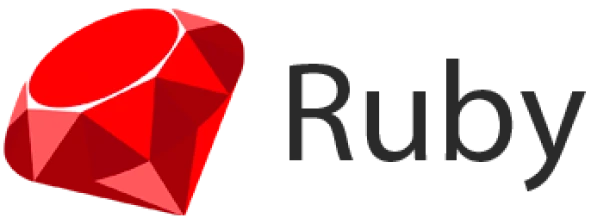


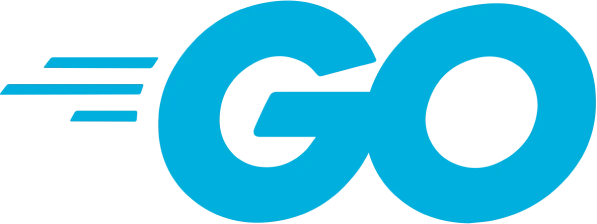

What is it like running Milvus on Tessell?
Ease of Consumption
Deploy fully-managed Milvus database based on choice of cluster shapes and cloud.
Select Cloud
Choose cluster shape
- Depending upon how many vectors you want to store.
- Chose from micro to large shape cluster based on requirements.
Deploy Cluster
.webp)
Self-healing and self-managed. 99.99% uptime
Build on the Milvus strength with reliability & resiliency built-in.
99.99% uptime SLA, and zero data corruption.
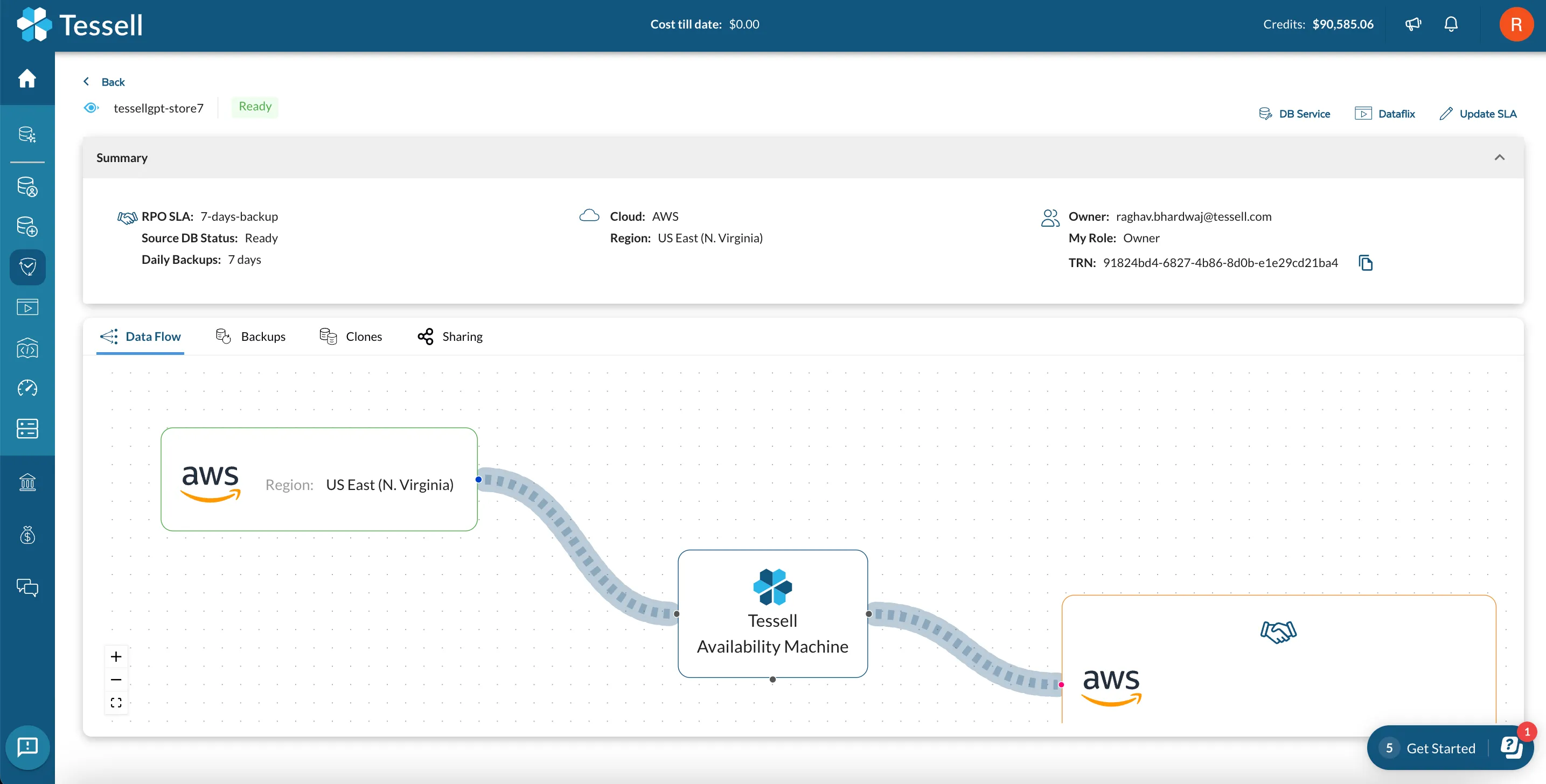
Collection & Indexes Lifecycle management
Support for database indexing and collection lifecycle management through Tessell on Milvus.
Powerful, flexible support for embeddings generated by LLMs.
Lightning-fast queries on any size data set
Cost-effective storage of vectors.
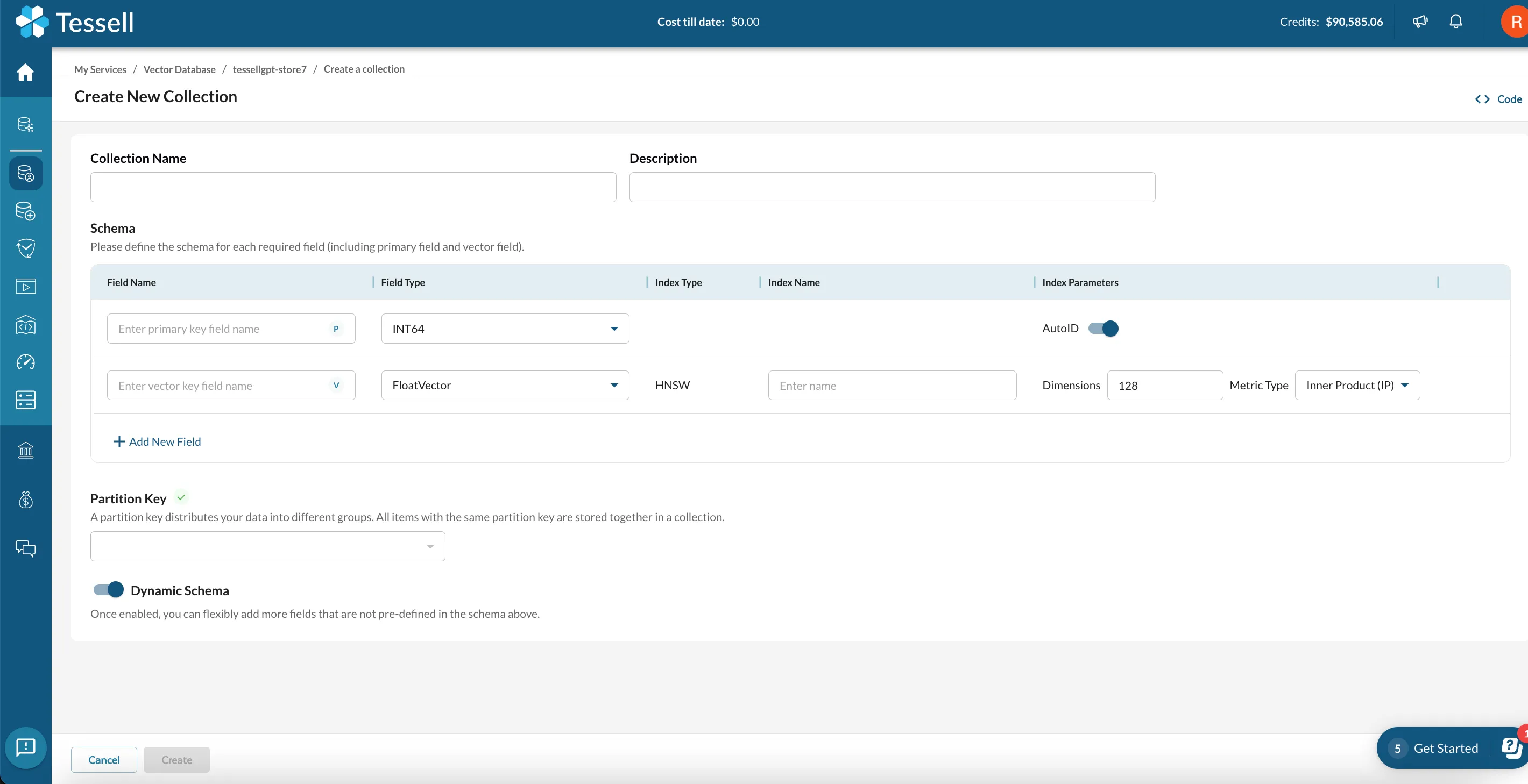